To do a study, I needed to record some temperature values from three points: outside, radiator and room. To build this I used the following main components:
– Arduino UNO
– SD Card module
– DS 1307 module
– Dallas 18B20 device
– DHT2302 device
– 1602 LCD Module
The data is recorded on an SD card and the real-time values of temperatures can be read on an LCD display.
The file from SD card can be open in Excel and with the results you can create chart.

The assembly scheme used was this:
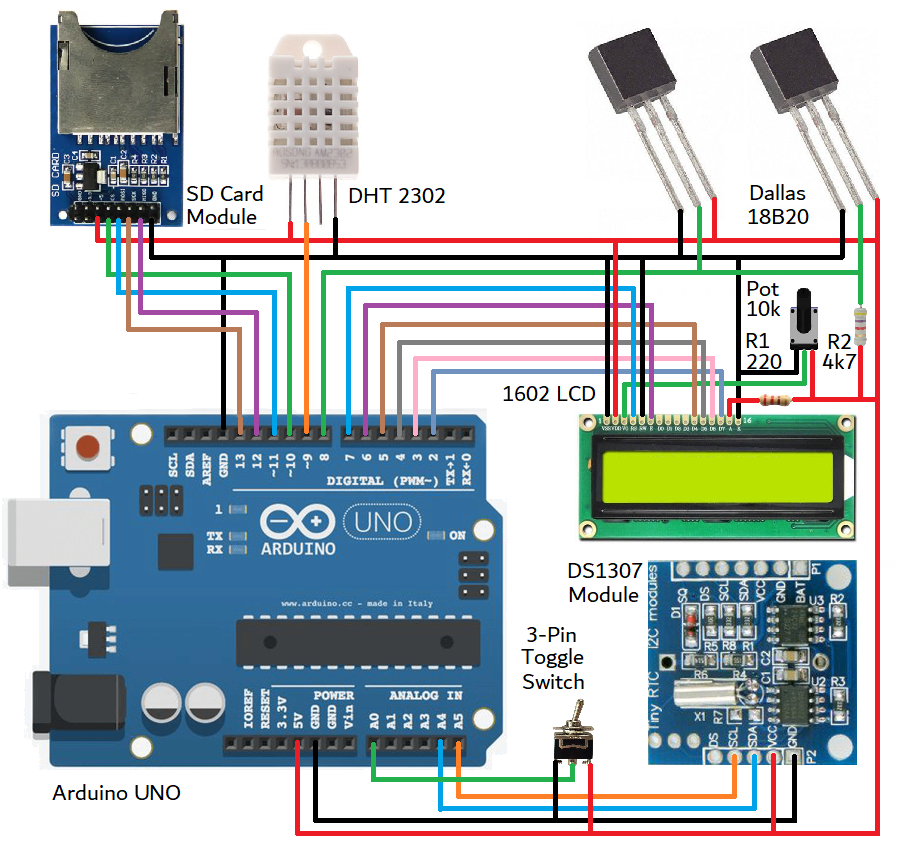
and the code loaded in an Arduino UNO was this:
#include <LiquidCrystal.h>
#include <Wire.h>
#include <RTClib.h>
RTC_DS1307 rtc;
#include <SD.h>
#include <DallasTemperature.h>
#define ONE_WIRE_BUS 8 //Data pins DS18B20 are connected to D8
OneWire oneWire(ONE_WIRE_BUS);
DallasTemperature sensors(&oneWire);
#include "DHT.h"
#define DHTPIN 9 // DHT222 to pin 9
#define DHTTYPE DHT22 // DHT 22 (AM2302)
DHT dht(DHTPIN, DHTTYPE);
char temp_str[10];
char rh_str[10];
int deviceCount = 0;
float T1, T2 ; //Variables for the 2 18B20
const byte FileOnOffButton = A0; //toggle switch (ON-ON) for starting and stopping the logging
bool WritingEnabled = false; //we switch the status of this with the buttons
bool SwitchStatus = false; //by default, this is false that means that the switch is OFF (no logging)
unsigned long startTime;
unsigned long elapsedTime;
int CS = 10; //chip select pin for the MicroSD Card Adapter, This is the CS Pin
File file; // file object that is used to read and write data
LiquidCrystal lcd(7, 6, 5, 4, 3, 2);
float t = dht.readTemperature();
const int chipSelect = 10;
int Temp = 0; // temprature
//##################
void setup() //
//##################
{
lcd.begin(16, 2);
dht.begin();
Serial.begin(9600);
sensors.setResolution(10); //10 bit resolution (0.25°C step)
// locate devices on the bus
Serial.print("Locating devices...");
Serial.print("Found ");
deviceCount = sensors.getDeviceCount();
Serial.print(deviceCount, DEC);
Serial.println(" devices.");
Serial.println("");
//Pin for the switch pins
pinMode(FileOnOffButton, INPUT);
//Serial.print("Initializing SD card...");
lcd.print("Initializing SD card...");
delay(1000);
lcd.clear();
pinMode(10, OUTPUT); // required for SD lib
digitalWrite(10,HIGH);
// see if the card is present and can be initialized:
if (!SD.begin(chipSelect))
{
// Serial.println("Card failed, or not present");
lcd.print("Card Failed or");
lcd.setCursor(0, 1);
lcd.print("Not present");
// don't do anything more:
delay(3000);
return;
}
//Serial.println("card initialized.");
lcd.print("Card Initialized.");
delay(2000);
lcd.clear();
Wire.begin();
rtc.begin();
if (! rtc.isrunning())
{
lcd.clear();
lcd.print("RTC NOT Running");
//Serial.println("RTC is NOT running!");
// following line sets the RTC to the date & time this sketch was compiled
// rtc.adjust(DateTime(__DATE__, __TIME__)); //useed when only to set date and time in firt setting of RTC
}
// rtc.adjust(DateTime(__DATE__, __TIME__)); use it when you need to correct the time
delay(2000);
}
//##################
void loop() //
//##################
{
lcd.setCursor(13, 0);
Temp = (5.0 * analogRead(A0) * 100.0) / 1024;
DateTime now = rtc.now();
// print to the serial port too:
//Serial.print(Temp);
//Serial.print(",");
//Serial.print(now.year(), DEC);
//Serial.print('/');
//Serial.print(now.month(), DEC);
//Serial.print('/');
//Serial.print(now.day(), DEC);
//Serial.print(' ');
//Serial.print(now.hour(), DEC);
//Serial.print(':');
//Serial.print(now.minute(), DEC);
//Serial.print(':');
//Serial.print(now.second(), DEC);
//Serial.println();
//lcd.setCursor(0, 0);
//lcd.print(Temp);
//lcd.print((char)223); // degrees character
//lcd.print("C");
lcd.setCursor(0, 0);
lcd.print(now.day(), DEC);
lcd.print('/');
lcd.print(now.month(), DEC);
lcd.print('/');
lcd.print(now.year(), DEC);
lcd.setCursor(11, 0);
if (now.hour() < 10) lcd.print("0"); // add zero
lcd.print(now.hour(), DEC); // Print hour
lcd.print(":");
if (now.minute() < 10) lcd.print("0"); // add zero
lcd.print(now.minute(), DEC); // Print minute
// lcd.print(":");
// if (now.second() < 10) lcd.print("0"); // add zero
// lcd.print(now.second(), DEC); // Print second
int s=now.second();
if(s==0)
{
//get the log toSD every 60 sec ( 1Min )
LogToSD(); //Log to SD using commands under void LogToSD()
}
delay(1000);
//Collect the values for each sensors
sensors.requestTemperatures(); //request the temperature
//Filling up the variables
T1 = sensors.getTempCByIndex(0);
T2 = sensors.getTempCByIndex(1);
//--DHT--
//float h = dht.readHumidity();
float t = dht.readTemperature();
//--DHT--
float humidity = dht.readHumidity(); // get the values from the DHT22
float temp = dht.readTemperature();
float convtemp = temp;
dtostrf(convtemp, 6, 2, temp_str);
if (isnan(temp) || isnan(humidity))
{
Serial.println("Failed to read from DHT");
lcd.clear();
}
else
{
Serial.print("Masuratori: ");
}
//Serial.print(elapsedTime); //time in ms
Serial.print("Sensor 1: ");
Serial.print(T1,2); //temperature, 2 digits (i.e. 28.12)
Serial.print(" ");
lcd.setCursor(0, 1);
lcd.print(T1,2);
Serial.print("Sensor 2: ");
Serial.print(T2,2);
Serial.print(" ");
lcd.setCursor(6, 1);
lcd.print(T2,2);
Serial.print("Sensor 3: ");
Serial.print(temp_str);
Serial.print(" ");
lcd.setCursor(11, 1);
lcd.print(temp_str);
Serial.println();
}
//##################
void LogToSD() //
//##################
{
DateTime now = rtc.now();
// open the file. note that only one file can be open at a time,
// so you have to close this one before opening another.
File dataFile = SD.open("datalog.csv", FILE_WRITE);
// if the file is available, write to it:
if (dataFile)
{
dataFile.print(T1);
dataFile.print(",");
dataFile.print(T2);
dataFile.print(",");
dataFile.print(temp_str);
//dataFile.print(",");
//dataFile.print(T4);
dataFile.print(",");
dataFile.print(now.year(), DEC);
dataFile.print('/');
dataFile.print(now.month(), DEC);
dataFile.print('/');
dataFile.print(now.day(), DEC);
dataFile.print(',');
dataFile.print(" ");
dataFile.print(now.hour(), DEC);
dataFile.print(':');
dataFile.print(now.minute(), DEC);
dataFile.print(':');
dataFile.print(now.second(), DEC);
dataFile.println(",");
dataFile.close();
}
// if the file isn't open, pop up an error:
else
{
//Serial.println("error opening datalog.txt");
lcd.clear();
lcd.print("File Error");
delay(2000);
}
}
This is real time temperatures displayed on the LCD:

And these are the data recorded from SD card to Excell :

I used Arduino IDE 1.8.1 version and the libraries shown in the code.